UWP and Xamarin Forms – How to display your app’s version number
Assume we want to automatically show the version number of your app in your UI, for example, the settings page or elsewhere. Your version number will normally be updated by your CI/CD system (updating Package.appxmanifest for UWP and AndroidManifest.xml for an Android Xamarin app).
Create a property to bind to
ViewModels and how they bind to your UI are out of scope for this post (as you’ll have already got this far). However, if you add a property to your view model like this:
public string VersionString
{
get
{
return "hello";
}
}
We can use this to test the binding before updating to the actual version number later.
Bind to it using UWP
For UWP you’ll need to use the TextBlock control and bind the Text property:
<TextBlock Text="{Binding VersionString}"></TextBlock>
Bind to it in Xamarin Forms
Xamarin Forms has a slightly different flavour, so you’ll need to use the Label control:
<Label Text="{Binding VersionString}"></Label>
Check the UI
You’ll see the following in your app:
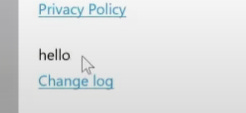
Updating the binding to show the version number
Because getting the version number differs by platform, you should use the super awesome Xamarin Essentials library.
Add nuget package to your Mobile class library and your UWP app following the documentation.
Then add the following using statement to the top of your ViewModel:
using Xamarin.Essentials;
And update your property code:
public string VersionString
{
get
{
return "Version " + AppInfo.VersionString;
}
}
That’s it! Xamarin Essentials handles the cross platform bits.
Confirm the version number is displayed
Relaunch your UWP app and you’ll see:
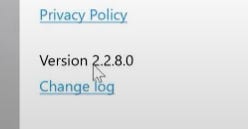
Launch the Xamarin app and you’ll see:
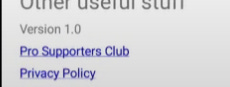
These values are pulled from Package.appxmanifest and AndroidManifest.xml respectively.
Demo video
Here is a nice YouTube Style™ video demo of the above: